Backup Plugin
Added in version 1.3.10.
The OctoPrint Backup Plugin comes bundled with OctoPrint (starting with 1.3.10).
It allows the creation and restoration [4] of backups of OctoPrint’s settings, data and installed plugins [5] (but doesn’t restore the same plugins versions [6]).
This allows easy migration to newly setup instances as well as making regular backups to prevent data loss.
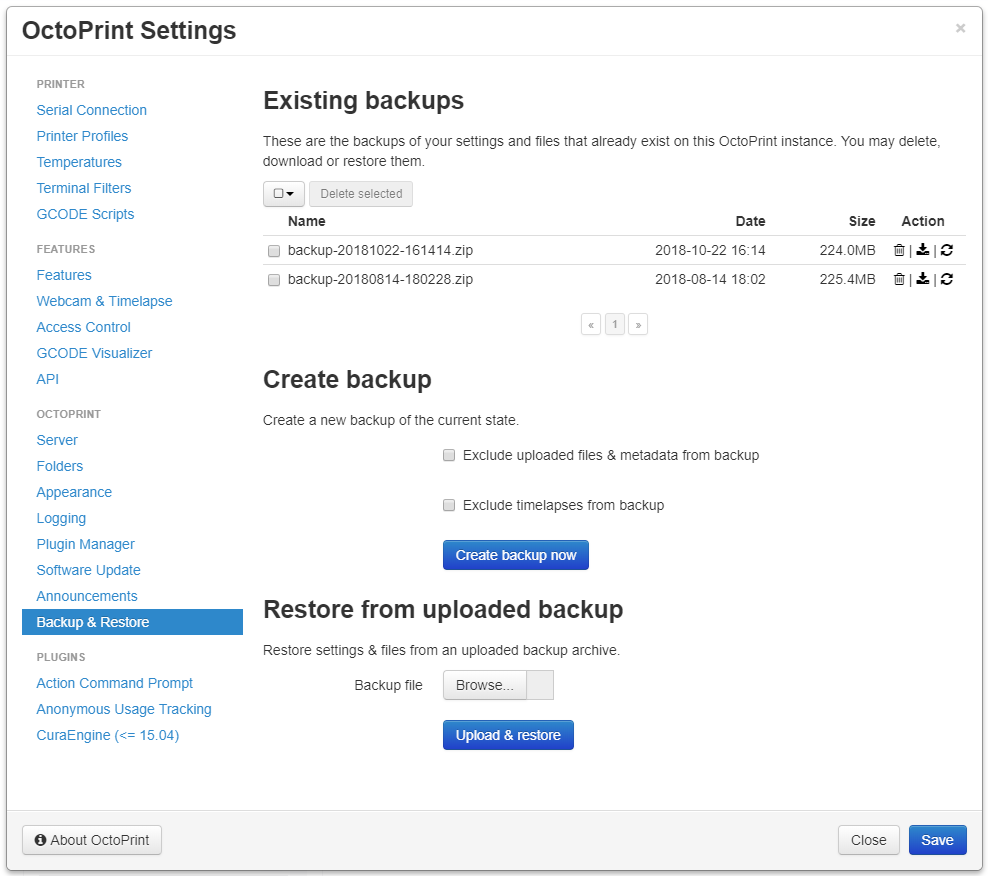
Fig. 14 The plugin’s settings panel with existing backups, the backup creation and restore sections.
As long as plugins adhere to the standard of storing their data and settings in OctoPrint’s plugin data folders, their data will be part of the backup. Note that the backups made by the Backup Plugin will not be part of any backups - you’ll need to persist the resulting zip files yourself!
Configuring the plugin
The plugin supports the following configuration keys:
restore_unsupported
: If the system you are installing OctoPrint on doesn’t support restoring backups or you want to disable that feature for other reasons, set this to true. Alternatively you can also set the environment variable OCTOPRINT_BACKUP_RESTORE_UNSUPPORTED to true. OctoPrint will then disable the restore functionality. Under normal circumstances you should not have to touch this setting (OctoPrint will do its best to autodetect whether it’s able to perform restores), thus it is not exposed in the Settings dialog.
Command line usage
The Backup Plugin implements a command line interface that allows creation and restoration of backups.
It adds two new commands, backup:backup
and backup:restore
.
$ octoprint plugins backup:backup --help
Initializing settings & plugin subsystem...
Usage: octoprint plugins backup:backup [OPTIONS]
Creates a new backup.
Options:
--exclude TEXT Identifiers of data folders to exclude, e.g. 'uploads' to
exclude uploads or 'timelapse' to exclude timelapses.
--help Show this message and exit.
$ octoprint plugins backup:restore --help
Initializing settings & plugin subsystem...
Usage: octoprint plugins backup:restore [OPTIONS] PATH
Restores an existing backup from the backup zip provided as argument.
OctoPrint does not need to run for this to proceed.
Options:
--help Show this message and exit.
Note
The backup:backup
command can be useful in combination with a cronjob to create backups in regular intervals.
Events
Events will not be triggered by CLI operations.
- plugin_backup_backup_created
A new backup was created. On the push socket only available with a valid login session with
Backup Access
permission.Payload:
name
: the name of the backuppath
: the path to the backupexcludes
: the list of parts excluded from the backup
Added in version 1.5.0.
Hooks
octoprint.plugin.backup.additional_excludes
- additional_excludes_hook(excludes, *args, **kwargs)
Added in version 1.5.0.
Use this to provide additional paths on your plugin’s data folder to exclude from the backup. Your handler also get a list of currently excluded sub paths in the base folder, so you can react to them. E.g. exclude things in your data folder that relate to uploaded GCODE files if uploads is excluded, or exclude things that relate to timelapses if timelapse is excluded.
Expects a list of additional paths relative to your plugin’s data folder. If you return a single ., your whole plugin’s data folder will be excluded from the backup.
Example 1
The following example plugin will create two files
foo.txt
andbar.txt
in its data folder, but flagfoo.txt
as not to be backed up.import octoprint.plugin import os import io class BackupExcludeTestPlugin(octoprint.plugin.OctoPrintPlugin): def initialize(self): with io.open(os.path.join(self.get_plugin_data_folder(), "foo.txt"), "w", encoding="utf-8") as f: f.write("Hello\n") with io.open(os.path.join(self.get_plugin_data_folder(), "bar.txt"), "w", encoding="utf-8") as f: f.write("Hello\n") def additional_excludes_hook(self, excludes, *args, **kwargs): return ["foo.txt"] __plugin_implementation__ = BackupExcludeTestPlugin() __plugin_hooks__ = { "octoprint.plugin.backup.additional_excludes": __plugin_implementation__.additional_excludes_hook }
Example 2
In this example the plugin will create a file
foo.txt
in its data folder and flag its whole data folder as excluded from the backup if uploaded GCODE files are also excluded:import octoprint.plugin import os import io class BackupExcludeTestPlugin(octoprint.plugin.OctoPrintPlugin): def initialize(self): with io.open(os.path.join(self.get_plugin_data_folder(), "foo.txt"), "w", encoding="utf-8") as f: f.write("Hello\n") def additional_excludes_hook(self, excludes, *args, **kwargs): if "uploads" in excludes: return ["."] return [] __plugin_implementation__ = BackupExcludeTestPlugin() __plugin_hooks__ = { "octoprint.plugin.backup.additional_excludes": __plugin_implementation__.additional_excludes_hook }
- Parameters:
list (excludes) – A list of paths already flagged as excluded in the backup
- Returns:
A list of paths to exclude, relative to your plugin’s data folder
- Return type:
octoprint.plugin.backup.before_backup
- before_backup_hook(*args, **kwargs)
Added in version 1.9.0.
Use this to perform actions right before a backup is created.
octoprint.plugin.backup.after_backup
- after_backup_hook(error=False, *args, **kwargs)
Added in version 1.9.0.
Use this to perform actions right after a backup was created or backup creation failed. The
error
parameter will be set toTrue
if the backup creation failed.- Parameters:
bool (error) – Whether the backup creation failed or not
octoprint.plugin.backup.before_restore
- before_restore_hook(*args, **kwargs)
Added in version 1.9.0.
Use this to perform actions right before a backup is restored.
octoprint.plugin.backup.after_restore
- after_restore_hook(error=False, *args, **kwargs)
Added in version 1.9.0.
Use this to perform actions right after a backup was restored or backup restoration failed. The
error
parameter will be set toTrue
if the backup restoration failed.- Parameters:
bool (error) – Whether the backup restoration failed or not
Helpers
The Backup plugin exports two helpers that can be used by other plugins or internal methods from within OctoPrint, without going via the API.
create_backup
- octoprint.plugins.backup.BackupPlugin.create_backup_helper(self, exclude=None, filename=None)
Added in version 1.6.0.
Create a backup from a plugin or other internal call
This helper is exported as
create_backup
and can be used from the plugin manager’sget_helpers
method.Example
The following code snippet can be used from within a plugin, and will create a backup excluding two folders (
timelapse
anduploads
)helpers = self._plugin_manager.get_helpers("backup", "create_backup") if helpers and "create_backup" in helpers: helpers["create_backup"](exclude=["timelapse", "uploads"])
By using the
if helpers [...]
clause, plugins can fall back to other methods when they are running under versions where these helpers did not exist.
delete_backup
- octoprint.plugins.backup.BackupPlugin.delete_backup_helper(self, filename)
Added in version 1.6.0.
Delete the specified backup from a plugin or other internal call
This helper is exported as
delete_backup
and can be used through the plugin manager’sget_helpers
method.Example The following code snippet can be used from within a plugin, and will attempt to delete the backup named
ExampleBackup.zip
.helpers = self._plugin_manager.get_helpers("backup", "delete_backup") if helpers and "delete_backup" in helpers: helpers["delete_backup"]("ExampleBackup.zip")
By using the
if helpers [...]
clause, plugins can fall back to other methods when they are running under versions where these helpers did not exist.Warning
This method will fail silently if the backup does not exist, and so it is recommended that you make sure the name comes from a verified source, for example the name from the events or other helpers.
- Parameters:
filename (str) – The name of the backup to delete
Source code
The source of the Backup plugin is bundled with OctoPrint and can be found in
its source repository under src/octoprint/plugins/backup
.